Given a Roman numeral, Write a code to convert roman to integer value.
Roman numerals are represented by seven different letters (I, V, X, L, C, D, M). These seven letters are used to make thousands of numbers.
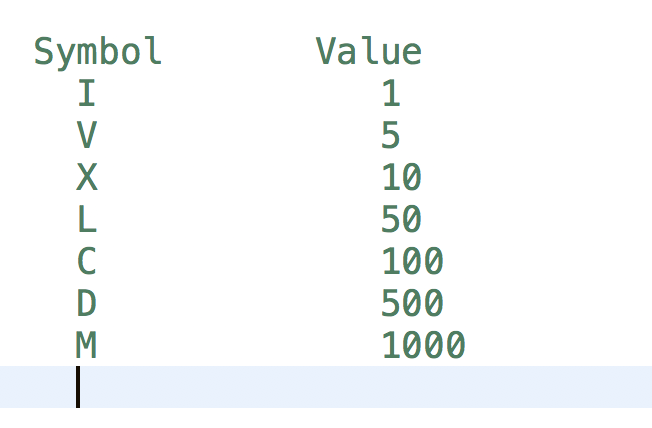
For example –
Example 1 –
Input : II , Output : 2
Example 2 –
Input : XI , Output : 11
Example 3 –
Input : IV , Output : 4
Example 4 –
Input : LVII , Output: 57
NOTE : The given input is guaranteed to be within the range from 1 to 3999.
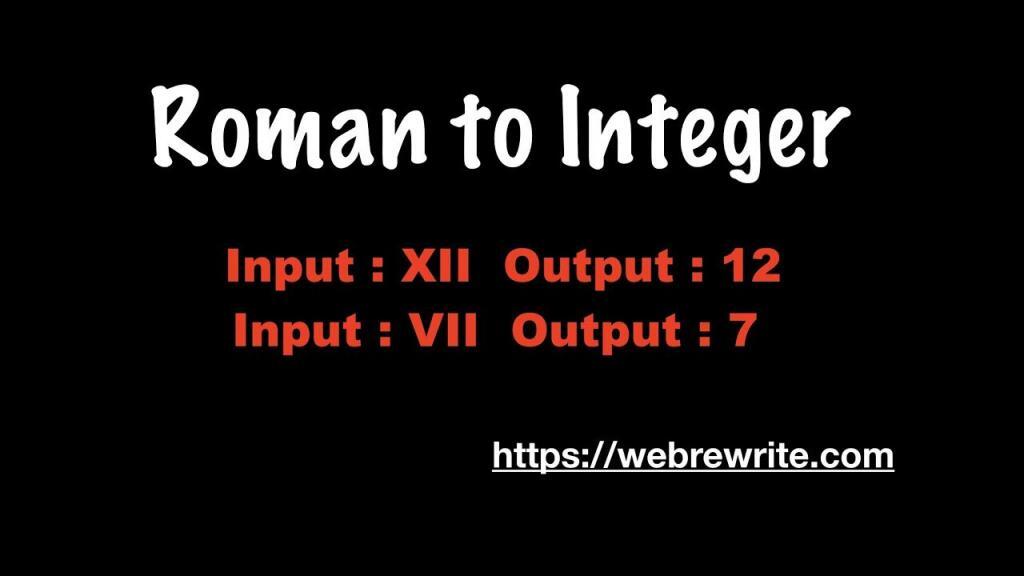
Programming Questions on Arrays
Programming Questions on Linked List
Programming Questions on String
We have discussed the problem statement. In this problem, we have to convert the roman number to an integer.
Convert Roman to Integer Value – Java Code
Before solving this problem, Let’s understand a few important points about Roman Numerals.
Roman numerals are usually written from largest to smallest from left to right. For example – XII (12), VII (7), LVII (57), etc.
However, the numeral for four is not IIII
. Instead, the number four is written as IV
. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as IX
. There are six instances where we need to do subtraction.
I
can be placed beforeV
(5) andX
(10) to make 4 and 9.X
can be placed beforeL
(50) andC
(100) to make 40 and 90.C
can be placed beforeD
(500) andM
(1000) to make 400 and 900.
Keeping these points in mind, here are the following steps to solve this problem.
- Traverse a string and pick one roman numeral (each character) at a time.
- Convert each symbol of Roman Numeral into the value it represents.
- If current value of numeral is greater than or equal to the value of next numeral, then add this value to the running total. Else subtract this value from the result.
The time complexity of this approach is O(n) and its space complexity is O(1).
convert roman numerals to numbers in java video tutorial
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
//Convert Roman Numerals to Decimal Value public class RomanToInteger { public static int convertRomanToInteger(String str) { //If string value is null or zero if(str == null || str.length() == 0) { return 0; } //Create a mapping of roman numerals and it's integer value Map<Character, Integer> charMap = new HashMap<>(); charMap.put('I', 1); charMap.put('V', 5); charMap.put('X', 10); charMap.put('L', 50); charMap.put('C', 100); charMap.put('D', 500); charMap.put('M', 1000); int result = 0; /* Traverse a string and pick each character at a time. */ for(int i = 0; i < str.length()-1; i++) { /* If current Roman numeral value is greater than then the next value, the do addition. */ if(charMap.get(str.charAt(i)) >= charMap.get(str.charAt(i+1))) { result = result + charMap.get(str.charAt(i)); } else { result = result - charMap.get(str.charAt(i)); } } //Add the value of last numeral result = result + charMap.get(str.charAt(str.length()-1)); return result; } public static void main(String[] args) { //String str = "IV"; //String str = "XXIV"; String str = "XC"; int result = convertRomanToInteger(str); System.out.println(result); } } |
Roman to Integer InterviewBit Solution
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
public class Solution { public int romanToInt(String A) { //If string value is null or zero if(A == null || A.length() == 0) { return 0; } //Create a mapping of roman numerals and it's integer value Map<Character, Integer> charMap = new HashMap<>(); charMap.put('I', 1); charMap.put('V', 5); charMap.put('X', 10); charMap.put('L', 50); charMap.put('C', 100); charMap.put('D', 500); charMap.put('M', 1000); int result = 0; /* Traverse a string and pick each character at a time. */ for(int i = 0; i < A.length()-1; i++) { /* If current Roman numeral value is greater than then the next value, the do addition. */ if(charMap.get(A.charAt(i)) >= charMap.get(A.charAt(i+1))) { result = result + charMap.get(A.charAt(i)); } else { result = result - charMap.get(A.charAt(i)); } } //Add the value of last numeral result = result + charMap.get(A.charAt(A.length()-1)); return result; } } |