Given a Roman numeral, Write a code to convert roman to integer value.
Roman numerals are represented by seven different letters (I, V, X, L, C, D, M). These seven letters are used to make thousands of numbers.
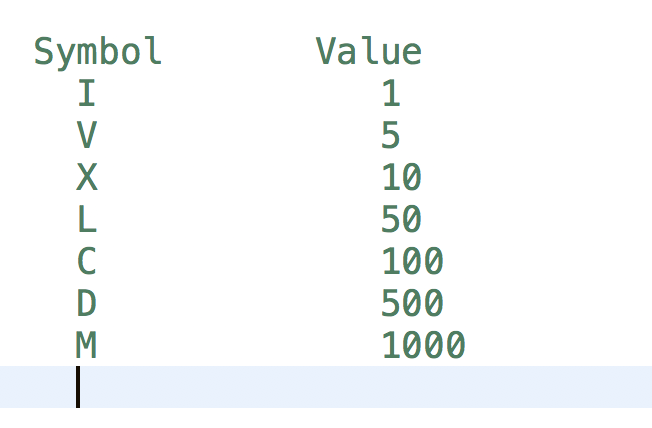
For example –
Example 1 –
Input : II , Output : 2
Example 2 –
Input : XI , Output : 11
Example 3 –
Input : IV , Output : 4
Example 4 –
Input : LVII , Output: 57
NOTE : The given input is guaranteed to be within the range from 1 to 3999.