In this tutorial, I am going to explain what are wrapper classes in java. Why it is introduced and the concept of autoboxing and unboxing.
Wrapper Class
As the name suggests, a wrapper class wraps (encloses) around a primitive data type and gives it an object appearance. In simple words, we can convert primitive data types into an object using their corresponding wrapper class.
Wrapper classes are final and immutable.
How to create immutable class in java
For example:
1 2 3 4 5 6 7 8 |
//int and double are primitive types int a = 5; double b = 6.75; //Converted into an object by using their respective wrapper classes Integer intObj = Integer.valueOf(a); Double dbObj = Double.valueOf(b); |
Primitive Data Type
A primitive data type defines the size and type of variable values. In Java, we have eight primitive data types, which are as follows:
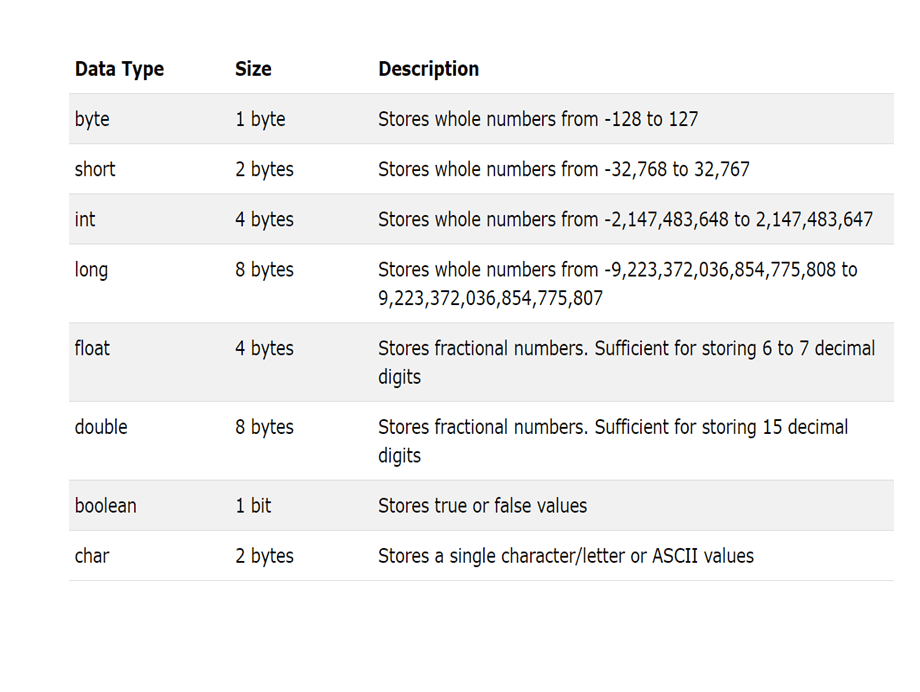
Now each primitive data type has its corresponding wrapper classes. These all classes are defined in java.lang package, hence we don’t need to import them manually.
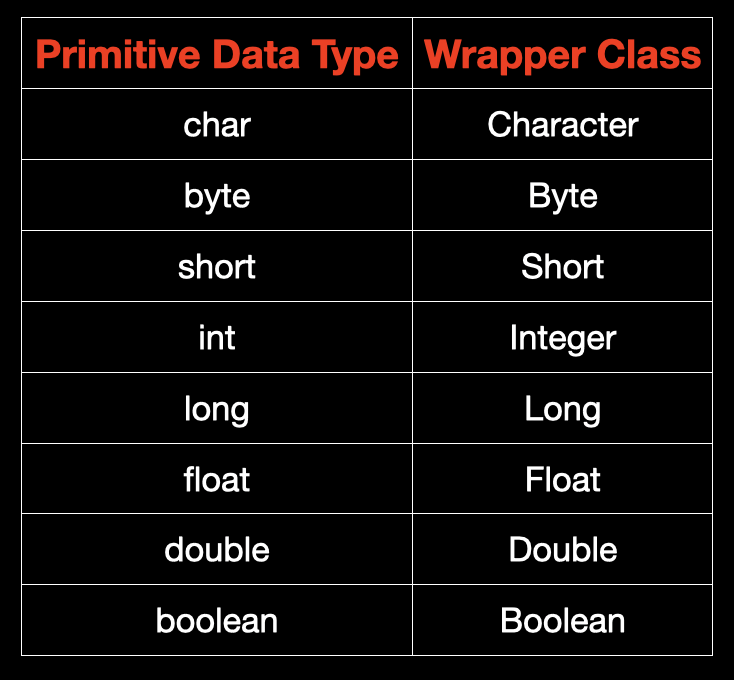
Why wrapper classes?
1. Java Collection Framework works only with objects.
For example:
1 2 3 4 5 6 7 8 9 10 |
//Invalid declaration, primitives are not accepted List<int> values = new ArrayList<>(); Stack<double> st = new Stack<>(); //Valid List<Integer> values = new ArrayList<>(); Stack<Double> st = new Stack<>(); |
Although, we can also pass primitive values. Then how does it work?
1 2 3 4 5 6 7 |
//primitive int a = 5; List<Integer> values = new ArrayList<>(); //works fine values.add(5); |
If a primitive value is passed then java converts the primitive value to its corresponding wrapper classes before storing it. This is called autoboxing.
How to convert primitive to object in java
Now the next question that comes to your mind is: how do we convert a primitive value to a corresponding wrapper class. For example, int to Integer or a double to Double?
We can convert the primitive value to its corresponding object by using a constructor or the static factory method.
For example –
1 2 3 4 5 |
//By using constructor of a wrapper class Double a = new Double(4.55); //By using static factory Double b = Double.valueOf(4.55); |
We should always use the static factory method over the constructor to convert the primitive values to objects. As the compiler does some performance optimization which is not possible if we use a constructor.
How to convert wrapper to primitive in java
If we want to convert objects into their primitive types, we can use the corresponding method such as doubleValue(), floatValue(), intValue() etc. The method is present in each wrapper class.
1 2 3 4 5 |
//Object Double a = new Double(5); //Convert to it's primitive value double b = a.doubleValue(); |
Autoboxing and Unboxing in Java
Let’s discuss the concept of autoboxing and unboxing that is related to wrapper classes.
Autoboxing is the automatic conversion of a primitive value into an object of the corresponding wrapper class.
For example –
When we add primitive value to a collection.
1 2 3 4 5 6 7 8 9 |
//primitive int a = 2; List<Integer> li = new ArrayList<>(); //Autoboxing li.add(a); |
The other example is to assign the primitive value to an object type.
1 2 3 |
Integer a = 6; Character ch = 'w'; |
In all these cases, internally java uses the valueOf() method to convert primitive to their corresponding wrapper class.
The java compiler converts the previous code to the following at runtime:
1 2 3 |
//Autoboxing by java compiler Integer a = Integer.valueOf(6) Character ch = Character.valueOf('w'); |
Unboxing on other hand is the automatic conversion of an object of a wrapper type into its corresponding primitive type.
1 2 3 4 5 6 |
List<Double> values = new ArrayList<>(); values.add(5.0); // 5 is autoboxed through method invocation. // Unboxing through assignment double val = values.get(0); System.out.println("val = " + val); |
Autoboxing and unboxing are done by the java compiler, we don’t have to do anything in it.